<Coding> with SonarPen
4 ways to use SonarPen in your own app or STEAM project
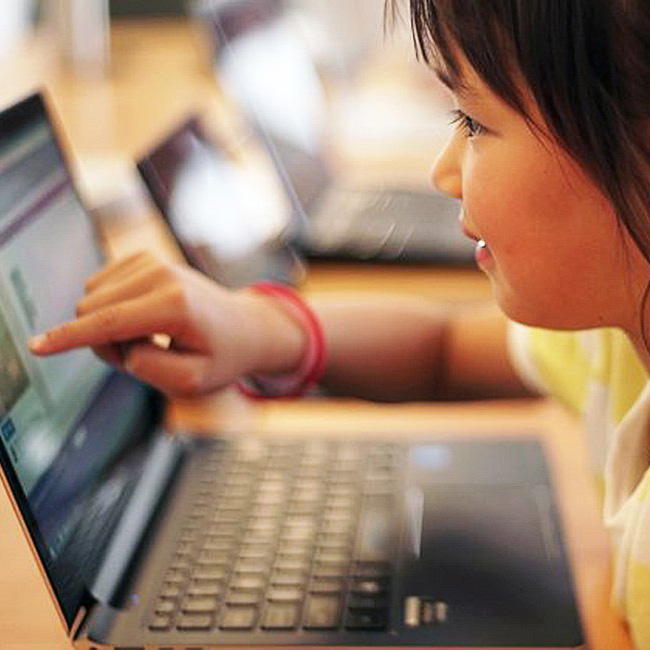
<Coding> with SonarPen
use SonarPen in your own app or steam project is very easy
This is a sample code to read SonarPen pressure and button in JavaScript. Plugin the SonarPen before running this code, make sure Windows Driver is not running at the same time.
Microphone access must be granted for SonarPen to work
SonarPen USB Type-C adatper or USB Type-C SonarPen is needed for the app to work
This is a sample code to read SonarPen pressure and button in Python. Plugin the SonarPen before running this code, and make sure Windows Driver is not running at the same time.
PyAudio library is used to produce sound to communicate with the SonarPen. It must be installed for the code to work. (available for Windows, MacOS and Linux)
SonarPen USB Type-C adatper or USB Type-C SonarPen is needed for the app to work
import pyaudio import numpy as np import threading import keyboard # Set the frequency of the tone frequency = 2000 # Set the sample rate sample_rate = 44100 # Generate the tone tone = (np.sin(2*np.pi*np.arange(sample_rate)*frequency/sample_rate)).astype(np.float32) # Initialize Pyaudio p = pyaudio.PyAudio() def play_tone(): # Open a stream stream = p.open(format=pyaudio.paFloat32, channels=1, rate=sample_rate, output=True) while True: try: # Play the tone stream.write(tone.tostring()) except Exception as e: print(e) break # Close the stream stream.stop_stream() stream.close() def record_amplitude(): # Open a stream for recording stream = p.open(format=pyaudio.paFloat32, channels=1, rate=sample_rate, input=True, frames_per_buffer=int(sample_rate * 0.01)) while True: try: # Record audio for 10ms data = stream.read(int(sample_rate * 0.01)) # Convert the data to a numpy array data = np.fromstring(data, dtype=np.float32) # Get the amplitude of the right channel amplitude = np.max(np.abs(data)) # Display the amplitude print("Amplitude:", amplitude) except Exception as e: print(e) break # Close the stream stream.stop_stream() stream.close() def playpause_button(): # detect button press while True: if keyboard.read_key() == "play/pause media": print("Button ======================================") # Start the threads print("If you are running this Pythono on a Windows PC, make sure SonarPen Windows Driver is not running or NO pressure will be detected.") play_thread = threading.Thread(target=play_tone) record_thread = threading.Thread(target=record_amplitude) button_thread = threading.Thread(target=playpause_button) play_thread.start() record_thread.start() button_thread.start()
Here is a two part demo that shows Scratch make use of SonarPen's pressure reading.
SonarPen USB Type-C adatper or USB Type-C SonarPen is needed for the app to work
SonarPen requires microphone to work, please allow use of microphone.
Stop the Scratch app before running another one.
Step 1. Calibrate SonarPen, detects MAX and MIN value of a SonarPen to be used in other Scratch app.
Step 2A. Plotter demo, enter the MAX, MIN value into the app, then change the movement of the plotter with pressure.
https://scratch.mit.edu/projects/806648708/
Step 2B. Draw! SonarPen remix, enter the MAX, MIN value into the app, then start drawing with pressure.
Step by step tutorial for integrating SonarPen in Scratch app is coming soon.
The SonarPen SDK is available for iOS and Android devices. We provide free to use license for app developers who like to integrate SonarPen in their free or pay app to be downloaded by general public in app stores. Please use the following form to tell use about your app idea.
This is a sample code to read SonarPen pressure and button in JavaScript. Plugin the SonarPen before running this code, make sure Windows Driver is not running at the same time.
Microphone access must be granted for SonarPen to work
SonarPen USB Type-C adatper or USB Type-C SonarPen is needed for the app to work
This is a sample code to read SonarPen pressure and button in Python. Plugin the SonarPen before running this code, and make sure Windows Driver is not running at the same time.
PyAudio library is used to produce sound to communicate with the SonarPen. It must be installed for the code to work. (available for Windows, MacOS and Linux)
SonarPen USB Type-C adatper or USB Type-C SonarPen is needed for the app to work
import pyaudio import numpy as np import threading import keyboard # Set the frequency of the tone frequency = 2000 # Set the sample rate sample_rate = 44100 # Generate the tone tone = (np.sin(2*np.pi*np.arange(sample_rate)*frequency/sample_rate)).astype(np.float32) # Initialize Pyaudio p = pyaudio.PyAudio() def play_tone(): # Open a stream stream = p.open(format=pyaudio.paFloat32, channels=1, rate=sample_rate, output=True) while True: try: # Play the tone stream.write(tone.tostring()) except Exception as e: print(e) break # Close the stream stream.stop_stream() stream.close() def record_amplitude(): # Open a stream for recording stream = p.open(format=pyaudio.paFloat32, channels=1, rate=sample_rate, input=True, frames_per_buffer=int(sample_rate * 0.01)) while True: try: # Record audio for 10ms data = stream.read(int(sample_rate * 0.01)) # Convert the data to a numpy array data = np.fromstring(data, dtype=np.float32) # Get the amplitude of the right channel amplitude = np.max(np.abs(data)) # Display the amplitude print("Amplitude:", amplitude) except Exception as e: print(e) break # Close the stream stream.stop_stream() stream.close() def playpause_button(): # detect button press while True: if keyboard.read_key() == "play/pause media": print("Button ======================================") # Start the threads print("If you are running this Pythono on a Windows PC, make sure SonarPen Windows Driver is not running or NO pressure will be detected.") play_thread = threading.Thread(target=play_tone) record_thread = threading.Thread(target=record_amplitude) button_thread = threading.Thread(target=playpause_button) play_thread.start() record_thread.start() button_thread.start()
Here is a two part demo that shows Scratch make use of SonarPen's pressure reading.
SonarPen USB Type-C adatper or USB Type-C SonarPen is needed for the app to work
SonarPen requires microphone to work, please allow use of microphone.
Stop the Scratch app before running another one.
Step 1. Calibrate SonarPen, detects MAX and MIN value of a SonarPen to be used in other Scratch app.
Step 2A. Plotter demo, enter the MAX, MIN value into the app, then change the movement of the plotter with pressure.
https://scratch.mit.edu/projects/806648708/
Step 2B. Draw! SonarPen remix, enter the MAX, MIN value into the app, then start drawing with pressure.
Step by step tutorial for integrating SonarPen in Scratch app is coming soon.
The SonarPen SDK is available for iOS and Android devices. We provide free to use license for app developers who like to integrate SonarPen in their free or pay app to be downloaded by general public in app stores. Please use the following form to tell use about your app idea.